Draggable Div with Pure JavaScript
HTML
JavaScript
Website
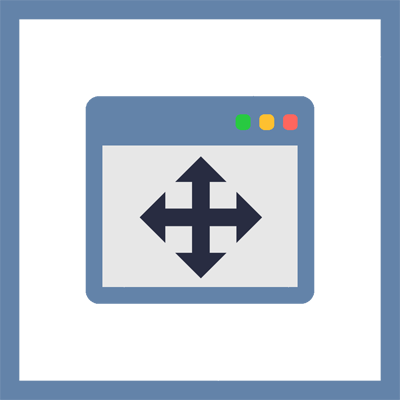
A JavaScript only solution to make a div draggable and that stays within the Viewport of the browser when dragging. No jQuery or other plugin is needed.
The code will put the div being dragged on top with the z-index. You can remove those pieces of code if you do not want it, or will only use one draggable div.
The downside is that this only works with a mouse, not a touch interface. If you wanto to use Touch Devices, check out my other solution that uses jQuery.
Code Snippets
var dragDivZindex = 100; //which div's to drag draggableDiv(document.getElementById("DraggableDiv1")); draggableDiv(document.getElementById("DraggableDiv2")); function draggableDiv(div) { //some variables var pos1 = 0 var pos2 = 0 var pos3 = 0 var pos4 = 0; var margin = 10; var extra_margin_right = 3; var extra_margin_bottom = 3; //set the inital z-index div.style.zIndex = dragDivZindex; //extra margin right when there is a vertical scrollbar if (document.body.scrollHeight > window.innerHeight) { extra_margin_right = 20; } //extra margin bottom when there is a horizontal scrollbar if (document.body.scrollWidth > window.innerWidth) { extra_margin_bottom = 20; } //use the Header for drag if there is one, if not drag on the whole div var header = div.id + '_Header'; if (document.getElementById(header)) { document.getElementById(header).onmousedown = dragDivMouseDown; } else { div.onmousedown = dragDivMouseDown; } //add a mouse enter event for the z-index div.onmouseenter = dragDivMouseEnter; //start dragging function dragDivMouseDown(e) { e = e || window.event; e.preventDefault(); //track coordinates pos3 = e.clientX; pos4 = e.clientY; //attach functions document.onmouseup = dragDivMouseUp; document.onmousemove = dragDivMouseMove; } //drag the div function dragDivMouseMove(e) { e = e || window.event; e.preventDefault(); //track coordinates pos1 = pos3 - e.clientX; pos2 = pos4 - e.clientY; pos3 = e.clientX; pos4 = e.clientY; var top = div.offsetTop - pos2; var left = div.offsetLeft - pos1; //check if the element does not exceed the viewport at the top of bottom if (top < margin) { top = margin; } else if (top + div.clientHeight + margin + extra_margin_bottom > window.innerHeight) { top = window.innerHeight - margin - div.clientHeight - extra_margin_bottom; } //check if the element does not exceed the viewport at the left of right if (left < margin) { left = margin; } else if (left + div.clientWidth + margin + extra_margin_right > window.innerWidth) { left = window.innerWidth - margin - div.clientWidth - extra_margin_right; } //set the css of the div div.style.top = top + 'px'; div.style.left = left + 'px'; } //stop dragging function dragDivMouseUp() { document.onmouseup = null; document.onmousemove = null; } //if there are more than one, set the element being dragged on top with z-index function dragDivMouseEnter() { dragDivZindex++; div.style.zIndex = dragDivZindex; } }
The CSS
.DraggableDiv { /* to allow the draggable div's to scroll with the content make the position absolute */ position: fixed; top: 30%; left: 20%; width: 250px; border: 1px solid #000000; background: #beccf3; box-shadow: 5px 5px 3px 0px rgba(97,97,97,0.5); } .DraggableDiv div { padding: 5px; } .DraggableDiv .Header { cursor: move; font-weight: bold; color: #ffffff; border-bottom: 1px solid #000000; background-color: #566895; } #DraggableDiv2 { cursor: move; top: 40%; left: 25%; }
The HTML
<div class="DraggableDiv" id="DraggableDiv1"> <div class="Header" id="DraggableDiv1_Header"> Header </div> <div class="Content"> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. </div> </div> <div class="DraggableDiv" id="DraggableDiv2"> <div class="Content"> <strong>No Header</strong> <br /> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. </div> </div>
Draggable Div 1
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Draggable Div 2 - No Header
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.